Important point, *easy to miss*: iphone.html is the code you need to be invoking from the browser, not index.html. It uses javascript to invoke index.html.
Huge gotcha: the code won't work unless you modify iphone.js to say "mydomain.com" instead of "jonathanstark.com".
Start with the posted html:
<html>
<head>
<title>Jonathan Stark</title>
<meta name="viewport" content="user-scalable=no, width=device-width" />
<link rel="stylesheet" href="iphone.css" type="text/css" media="screen" />
<script type="text/javascript" src="jquery.js"></script>
<script type="text/javascript" src="iphone.js"></script>
</head>
<body>
<div id="header"><h1>Jonathan Stark</h1></div>
<div id="container"></div>
</body>
</html>
Remember, this is iphone.html. It runs a javascript which loads index html.
Copy the posted code into "iphone.css". It's similar to chapter 2.
Now, create iphone.js with this code:
$(document).ready(function(){
loadPage();
});
function loadPage(url) {
if (url == undefined) {
$('#container').load('index.html #header ul', hijackLinks);
} else {
$('#container').load(url + ' #content', hijackLinks);
}
}
function hijackLinks() {
$('#container a').click(function(e){
e.preventDefault();
loadPage(e.target.href);
});
}
This does two things - first, it copies the area specified by the tags (either #header ul, or #content) into the #container section of iphone.html. It then turns every link into copied into a javascript function.
Note that there are no links to be copied in the #content section of the links, so there will be no links displayed except those from index.html.
If you run it in the iPhone simulator, it looks like this:
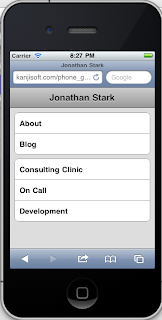
Next, get the "image loading" effect. Add this to the first line of the "loadPage" function:
$('body').append('Loading...');
And this to the end of the hijacklinks function:
$('#progress').remove();
When the page loads, you should see a little "Loading..." just below the "Jonathan Stark" header, which should disappear when the page shows up.
The next step is to add the css to make it look prettier...
#progress {
-webkit-border-radius: 10px;
background-color: rgba(0,0,0,.7);
color: white;
font-size: 18px;
font-weight: bold;
height: 80px;
left: 60px;
line-height: 80px;
margin: 0 auto;
position: absolute;
text-align: center;
top: 120px;
width: 200px;
}
The radius rounds the corners of the the box. the background color makes it black with an alpha (transparency) of 70%. The text color is white, it's 60 px from the left. Not sure what the purpose of line-hight and margin are, but they work. Now you should get a nice black box with white text showing up when the page loads.
There's a gotcha in the the next cool trick, which is to take each page's h2 and make the page's h1. Don't be fooled by the use of the word "title", he doesn't mean the html title. You do this by adding these lines just before the progress indicator is removed:
var title = $('h2').html() || 'Hello!';
$('h1').html(title);
$('h2').remove();
Note that if there's no h2, it will show "Hello". You shouldn't be getting that, but I think you might. I did.
Note that it will replace the previous h1 with the old h2, and get rid of the old h2.
This is what you should get. You can see it clearly in the before after at:
http://ofps.oreilly.com/titles/9780596805784/ch03_id35816678.html#beforeMovingHeadingToToolbar
and
http://ofps.oreilly.com/titles/9780596805784/ch03_id35816678.html#afterMovingHeadingToToolbar
See how the about moved up to replace "Jonathan Stark"?
However, if you get the hello, as I did, don't be shocked. I think the problem is that the initial invocation of iphone.html is stays in effect through the substitute command - index.html never gets called. And there is no h2 in iphone.html. The rest of the the links get transferred to by use of the loadPage(e.target.href);
loadPage(e.target.href);
To prevent this, you could do something like set a hidden variable the block that checks for the empty url, and check it later to see of you're on the first page, and if so account for that in the move logic. But, in the interests of moving on, we'll leave it at "hello". It works fine on the linked pages.
The next step is adding an elipse. I'm kind of off track again, because it looks like he's working from the chapter 2 linked pages. But, this is just to demonstrate wrapping, so I'll change the "Consulting Clinic" header to "A Consulting Clinic with an Edge for Your Company"
That leave an image that looks like this:
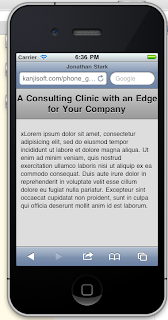
Add the ellipsis by adding this to the #header ht in the css:
max-width: 160px;
overflow: hidden;
white-space: nowrap;
text-overflow: ellipsis;
Max width is just what it says - a max width. The overflow:hidden means "chop off" and content extend beyond the (max) width. white-space: nowrap prevents wrapping, and of course text overflow ellipsis tells it to create an ellipsis in an overflow situation. Let's check it out:
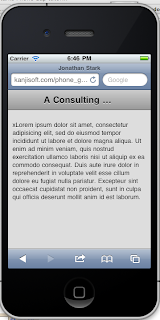
Cool.
The next step is to add this:
scrollTo(0,0);
The reason is, if you are somewhere on a page, and you click a link to get to another page, it will take you to the same section of the new page as the old. Well, it's the same page, iphone.html, and stark suggests this is the reason - you're not going to a new page. The javascript keeps you on the same page. To make this less egregious, you put the scrollto(0,0) command in.
To test this, we could try throwing a bunch of text into a couple of pages, iphone.html and consulting_clinic.html.
So, before we make the change, the first page load shows this:
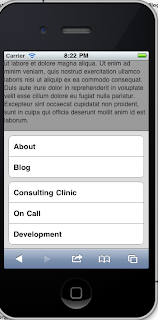
When we link, it goes to the same area of the "new" but really the same page:
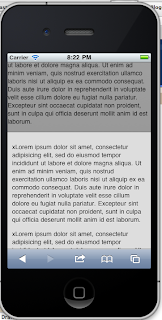
And finally after in inserting the (scroll 0,0), and going through the same sequence, we see it's gone to the top of the page:
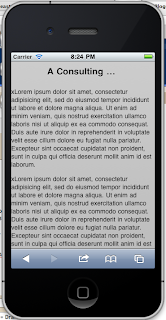
We'll wrap this up tomorrow.
No comments:
Post a Comment