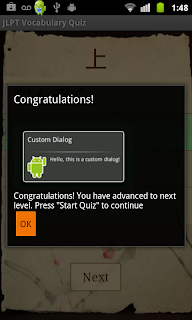
Four things jump out immediately
1. Center the button
2. Get rid of the ugly orange color of the button
3. The "Congratulations" on the top is redundant
4. We need a better image.
Ok, on centering, in android, there are two kinds of centering, one for the view itself, and one for the text in the view. I've got them both, so, let's choose one from the project.
Let's try this:
android:layout_gravity="center_vertical|center_horizontal"
Fix the title while we're at it:
dialog.setTitle("");
And test it.
Ok. But, it's quickly apparent we need to center the image also
Ok, did that and adjusted the text a bit.
Now, lets's set the image. Let's find that CustomDialog tutorial on Google.
Here it is a couple of posts ago:
http://developer.android.com/guide/topics/ui/dialogs.html#CustomDialog
So, it's under Dialog, just look for CustomDialog.
And here it is:
image.setImageResource(R.drawable.android);
Ok, all I really needed to do was look at the code.
Let's set the image to one of the Lin Golden's amazing works, "Looking at the Moon-Niou"
http://www.flickr.com/photos/purestgold/4741593238/sizes/m/in/set-72157624240358587/
Hmmm; it doesn't show. Let's try making a .png (it's currently jpg).
Ok, not bad. But the image is too large - it takes up the whole page. The button is really shrunken down. Let's try a different size.
240 x 169.
Ok, that's better:
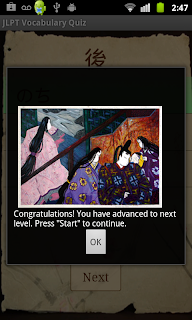
There are still some issues. Why is the background so dark? It basically black out the whole display, making some what disorienting and jarring. Also, the image is still off to the left a bit for some reason. Also, I need to identify the image and possibly create a link. Also, I need to vary the images; I would like to rotate about 10 of them. I also need to figure out how to handle the toast dialog, if they don't get all of them right. I think I'll just leave that as a toast.
There are a couple of other usability issues. You need to press this tiny button to get to the original display. Then, you have to press another button. Also, the dialog jumps out at you; I would rather a slower fade in.
I really don't like the double-touch to get to the next level. But, if I get rid of the button click, I'm back to my original problem of the dialogue immediately disappearing.
What if I make it an activity instead of a dialog? Let's to a quick test.
Add this:
import android.app.Activity;
import android.os.Bundle;
public class NextLevel extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.next_level);
}
}
Then call the activity:
// pass control to activity
Intent i = new Intent(QuestionActivity.this,
NextLevelActivity.class);
startActivity(i);
Comment out the show dialog.
//showDialog(NEXT_LEVEL_ID);
Then, put the finish back in,
finish();
And see if the activity display hangs up there.
Ok, I haven't loaded up the image or anything, so all I'm getting is the display button. How do lookup image? Is it a view?
Here's a tutorial:
http://www.higherpass.com/Android/Tutorials/Working-With-Images-In-Android/
ImageView image = (ImageView) findViewById(R.id.test_image);
Actually, I can probably take it right from the dialog code:
CharSequence text = "Congratulations! You have advanced to next level. Press \"Start\" to continue.";
TextView nextLevelText = (TextView) dialog
.findViewById(R.id.next_level_text);
nextLevelText.setText(text);
ImageView image = (ImageView) dialog.findViewById(R.id.image);
image.setImageResource(R.drawable.a);
Actually, we need to pull the dialog and let the find by view work from activity.
Ok, well, since I've set the style of the display to Dialog in the manifest,
It now looks exactly the same as the dialog previously shown. Cool :)
Now, let's see if I can kill it with the timer...
Here's some timer code:
private CountDownTimer mCountDownTimer = null;
mCountDownTimer = new CountDownTimer(totalMsecs, callInterval) {
public void onTick(long millisUntilFinished) {
float fraction = millisUntilFinished / (float) totalMsecs;
// progress bar is based on scale of 1 to 100;
m_bar.setProgress((int) (fraction * 100));
}
public void onFinish() {
handleTimeOut();
}
}.start();
We'll modify it to only do something on finish:
CountDownTimer countDowntimer = new CountDownTimer(totalMsecs, callInterval) {
public void onTick(long millisUntilFinished) {
}
public void onFinish() {
NextLevelActivity.this.finish();
}
}.start();
And set up the params like this:
totalMsecs = 1 * 2000; // 2 seconds
callInterval = 1000;
Call it a couple of time just to make sure it doesn't hang or something.
Nice! it works. We can get rid of the variable declaration:
new CountDownTimer(totalMsecs, callInterval) {
public void onTick(long millisUntilFinished) {
}
public void onFinish() {
NextLevelActivity.this.finish();
}
}.start();
And get rid of the button and the "click next" text.
And heres what we get:
Well, for some reason it's not uploading properly right now. But, the main thing is, we got rid of the extra button push, and the clutter of the button on the display.
That's a wrap for now!
No comments:
Post a Comment